Getting Started with Julia Lang
During my early studies and later in my career I had the opportunity to hone my engineering skills in a variety of different programming languages. I started in my early teenage years with QBasic and then progressed on to more advanced languages.
In open source projects I've largely worked on JavaScript (node.js) and PHP codebases, while my professional career has taken me to Java, Ruby (on Rails) and finally Python. Little bits of exposure left and right to newer languages like Go and Rust, but the above are the main languages I've done actual work in.
Over the years I have worked on a number of optimization problems that required the use of machine learning algorithms and libraries or the plotting of graphs. The go-to language for this has naturally been Python with its large collection of data science packages of the like of numpy, scipy, matplotlib and not to mention pytorch and tensorflow.
Now there's nothing wrong with any of the above. Python is a solid language with a long history and its grown to become the language of choice for data science. For me though, Python never felt quite right. The Zen of Python makes you think the language is opinionated and has clear structure to it. Oftentimes I found the opposite to be true. Where in Ruby (on Rails) "convention over configuration" rules the thinking Python leaves most up to the developer.
Popular web frameworks like Django and Flask come with short examples on how to build an application but leave a lot unanswered when your application starts growing. Where do routes live? Where do I put my models? As a result every project will look differently making it inherently difficult to jump into new codebases.
In a similar vein Python has the basic building blocks for OOP and functional programming, but stops there. Private variables don't really exist, static methods and class methods require the use of decorators, lambda is but a crippled version of implementations in other languages like Ruby and JavaScript, map
is a built-in function while reduce
was demoted to the functools
package. Even though the language technically supports these paradigms you will have to look hard to find them embraced in the popular packages.
Now you could argue that I'm comparing apples with oranges and to some extend I am. After all I'm comparing frameworks and not languages. To me though both go hand in hand. You can't have a language without the packages that make up said language. Just like a human language is influenced and evolves through its speakers, a programming language and the culture around it is defined by its community and ecosystem.
To be clear though - preferences differ and I'm not arguing that Python is a bad language, quite the contrary, it has built up a large following due to it being easy to learn and having a large ecosystem. It's just not a language that satisfies me because I care about structure and ultimately that's what made me look elsewhere.
Meet Julia
Julia goes back to 2009 and was started to create a fast high-level language. It wasn't until 2012 for Julia to be first announced and publicly released and version 1.0 landed 2018.
Julia has a dynamic type system, built-in package manager, multiple dispatch, performance approaching that of C and an ability to natively interface with other languages like Python. It's designed for distributed computing and makes it trivial to run algorithms on GPUs.
The ability to natively and easily interface with other languages sticks out to me. Take a look at the following example which uses PyCall to call some Python code from within Julia:
using PyCall
math = pyimport("math")
math.sin(math.pi / 4) # returns ≈ 1/√2 = 0.70710678...
The beauty is that you can very easily mix Python and Julia this way. Python's entire ecosystem including all its great libraries are available while you can also explore Julia's ecosystem. In fact Plots.jl, a plotting library for Julia can use PyPlot as one of its backends.
A neat feature of Julia is that you can use LaTeX shortcuts in the editor. For example when you type \sqrt<TAB>
most Julia editors and the Julia REPL will automatically turn this sequence into the square-root sign: √. In this case √ is also aliased to the sqrt
function. This makes Julia great for mathematical applications where you can use mathematical notation in your programs.

Julia lends itself to scientific applications and machine learning. Tools like Flux.jl
While it's true that Julia is heavily used in research it doesn't mean that you can't also build run-of-the-mill web applications. Frameworks like Genie
Installing Julia
The easiest way to install Julia is to download the compatible package for your OS on the official website. Personally I tend to use asdf-vm as it allows me to install and run multiple versions of my favourite languages simultaneously.
With asdf-vm installing Julia becomes as easy as:
$ asdf plugin add julia
$ asdf install julia 1.6.0
REPL
Julia's REPL is different from what you may be used to from other languages. It comes bundled with a package manager and several different modes.
The main shortcuts that you'll want to remember are:
]
gets you into package mode, from here you canadd
orupdate
packages and manage projects?
gets you into help mode. Julia is a well documented language and the help mode will allow you to find explanations and examples for all the built-ins;
is how you can enter shell mode allowing you to use a bash-like shell without leaving the Julia interpreter
Installing packages
Installing packages is straight forward. Press ]
to go into package mode and then add $PackageName
to install a package named $PackageName. Note that this will install packages in the globally.
A package you'll definitely want to install globally is called Revise.jl.
Revise.jl
Revise allows you to hot reload code while working in the REPL. This is especially useful in Julia since the REPL takes a little longer to start than in other languages. With Revise you can edit code in your IDE and have it reflected in the REPL immediately.
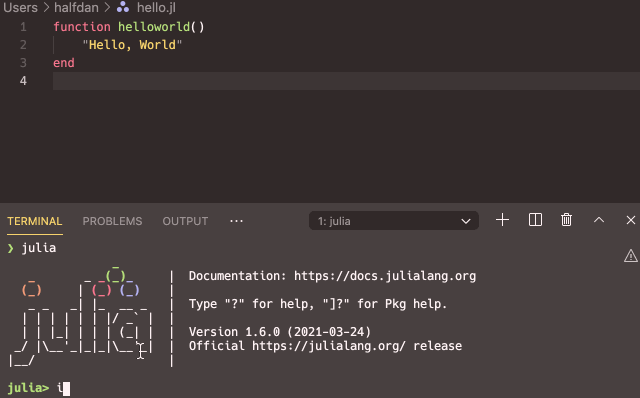
Editors
The two main editors for Julia are Juno and Julia for VSCode. Julia for VSCode is relatively young and was introduced at the last JuliaCon in 2020. Juno is based on Atom which has seen some decline in usage over time.
Since I'm already using VSCode for all my other development using the Julia for VSCode extension was an easy decision. It has all the features present in Juno.
Conclusion
Julia is a fascinating language with a growing community and a solid package ecosystem. While the ecosystem isn't as vast as Python's, it is trivial to call out to Python, R, C and other languages.
Julia's package manager is fast, easy to use. It's a breath of fresh air when compared to the shenanigans one has to deal with in Python with pip, setuptools, conda, poetry, et.al.
The lack of object-oriented programming features is hardly noticeable once you are comfortable with multiple dispatch. In fact Julia's simplicity and well thought out type system make it easy to reason about the objects you're dealing with in code. On top of that, I find Julia very easy to learn and well positioned to attract developers, data scientists and researchers who traditionally picked Python.